Card Game
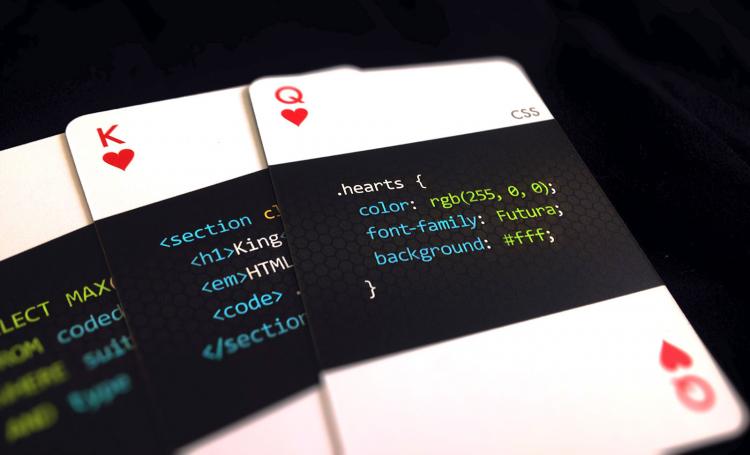
Goal:
Create a usable deck of cards using enums and implement the rules for a card game.
Steps:
- Create an enum for Suit. A Suit refers to the four suits of a standard card deck: Spades, Clubs, Hearts, and Diamonds.
- Create an enum for Rank. Rank refers to all possible values for cards within the suits: Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, Jack, Queen, King, and Ace.
- Create an int member variable for Rank called value and a getter to access it.
- Give appropriate default values for each Rank e.g. for Blackjack you might have 2 for Two, 10 for a face card(Jack, Queen, King), and 11 for an ace. Don't forget to initialize value in a Rank constructor.
- Create a method in the CardDealer class that initializes the deck Member variable and populates it with every Card combination of Rank and Suit so it becomes a complete 52 card playing deck. Hint: Use nested for loops and the .values() method of your enums.
- Create a shuffle method that rearranges the deck into a random order.
- Write code that would enable you to simulate a card game of your choice that uses a standard deck of playing cards. Note: You may create any additional classes, methods or variables that might be helpful to you. Depending on your game you may also need to tweak the default values of your Ranks.