Snake - Part 3
Now we will get the snake to grow as it eats the food. You will use an ArrayList to hold all the segments of the snake because ArrayLists can grow as new elements are added.
1. More Game Variables
In the Game Variables section of the code, create and initialize an ArrayList of Segments. (This will be your snake tail!)
2. Tail Management Methods
drawTail()
- Add code to draw a 10 by 10 rectangle for each Segment in your snake's tail.
manageTail()
- Call the checkTailCollision method
- Call the drawTail method
- To make the tail "move" towards the new location of its head, add a new segment to the tail with the same x and y values as the head segment.
- Remove the first segment from the tail, so the tail stays the same length
checkTailCollision()
If the snake crosses over its own tail, the game resets. So....If the snake head is in the same location as one of its tail segments:
- Set the count of food eaten back to 1.
- Reset the tail to a new empty ArrayList
- Add a new segment to the tail that has the same x and y as the snake's head
drawSnake()
Call manageTail from the drawSnake method after the head has been drawn.
eat()
When food is eaten, add a new segment to the tail, that has the same x and y as the snake's head.
3. Testing
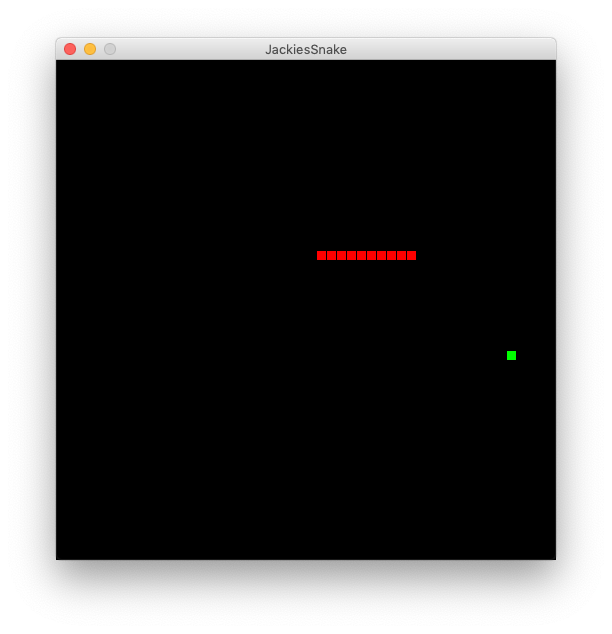
Now run your code to see if it works.
- Test that the tail grows when food is eaten
- Test that the snake reappears on the opposite side of the game when it should
- When the tail is long enough, test the snake shrinks when the head crosses the tail