Snake - Part 2
Now we will get the snake to move around, change direction when the arrow keys are pressed, and grow when it eats the food.
1. More Game Variables
Find the Game Variables section of the starter code.
- Create and initialize an int variable to hold the direction of your snake. Initialize this variable to UP ( a Processing constant ).
- Create and initialize an int variable to hold how many pieces of food the snake has eaten.
2. Control Methods
move()
- Complete the if-else if statement provided in the starter code and make sure it uses your direction variable.
- Call the checkBoundaries method after the switch statement to make sure the snake doesn't go off the screen.
- Call the move method in the draw method, before drawSnake().
checkBoundaries()
- Add code to this method to check if the snake's head is out of bounds (if it is, teleport your snake to the opposite side).
keyPressed()
- Add code to this method to set the direction variable to the keyCode of the key that was pressed. Hint: To understand this better, check it out in the Processing Reference .
- Make sure that the key for your current direction’s opposite doesn’t work (ex. if you’re going up, the down key shouldn’t have any effect)
eat()
Add code to this method to check if the head is in the same spot as the piece of food. If it is, the food must be eaten as follows:
- Increment the variable that keeps track of food eaten
- Drop a new piece of food for the snake to find.
Call the eat method at the end of the draw method, after drawSnake().
3. Testing
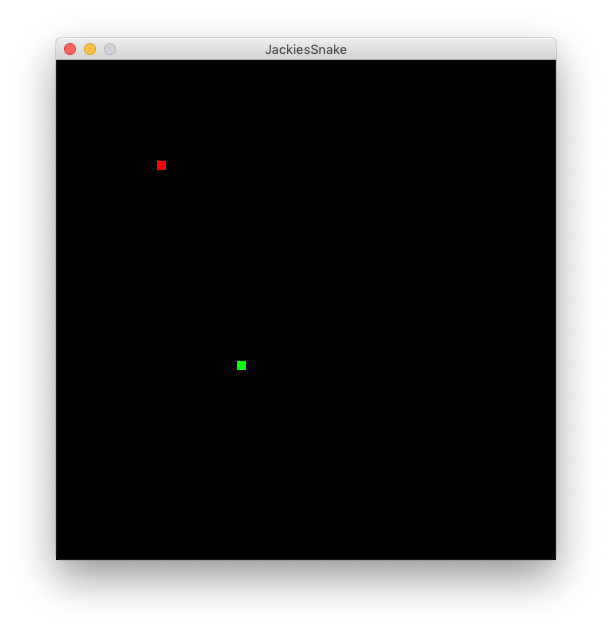
Now run your code to see if it works.
- The "snake" should now move and change direction when the arrow keys are pressed (within the rules described above).
- Test that the snake reappears on the opposite side of the game when it should
- If the snake moves over the food, a new piece of food should be drawn.