Snake
In this game, the player controls the movement of a snake. The snake is made up of a collection of Segments. Each Segment is drawn as a 10 by 10 square. Snake food is also square, and as the snake eats the food, it grows an additional Segment. More food then appears in a new location.
1. The Segment Class
Find the Segment class in the starter code.
- Add x and y member variables. They will hold the corner location of each segment of the snake.
- Add a constructor with parameters to initialize each variable.
2. Game Variables
Find the Game Variables section of the starter code.
- Create (but do not initialize) a Segment variable to hold the head of the Snake.
- Create (but do not initialize) foodX and foodY variables to hold the location of the food.
3. Settings Methods
settings()
Set the size of your sketch (default: 500 by 500).
4. Setup Methods
setup()
- Initialize your head variable to a new segment.
- Use the Processing frameRate(int rate) method to set the rate to 20. Go to the Processing Reference for a full explanation of what this method does.
- Call the dropFood() method.
dropFood()
In the dropFood method, set the x and y values of the food to a random location in the game. Hint: Since the food is 10 by 10, if the screen width is 500, you could use the following code to initialize the x variable:
foodX = ((int)random(50)*10);
5. Draw Methods
draw()
- Set the background to a solid color
- Call the drawFood and drawSnake methods.
drawFood()
- Draw a piece of food that is a 10 by 10 square. (Use the food variables you declared earlier)
- Make it an interesting color
drawSnake()
- Draw the snake's head. Hint: Draw a rectangle using the head's co-ordinates.
- Make it a different color from the food so you can tell which is which.
5. Testing
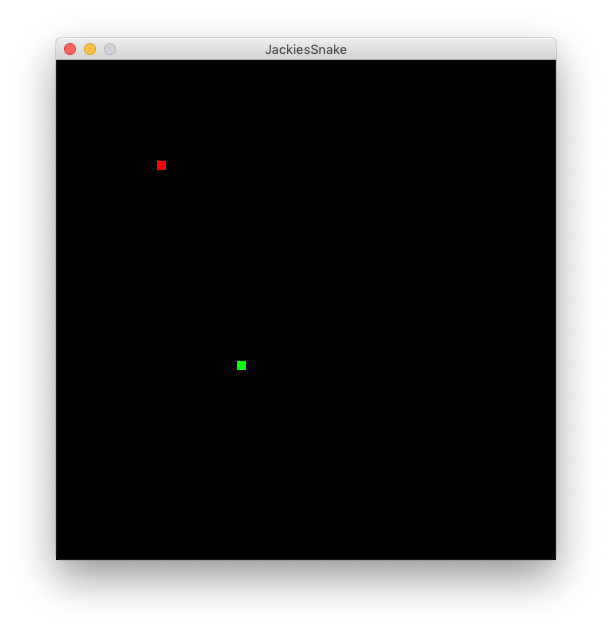
Now run your code to see if it works so far. You should see one square for the snake's head and another for the food.