Game Loop
Timer
A Timer will control the rate at which the game is refereshed or repainted, also known as the frame rate (or speed) of the game. The following steps will create and start the timer as part of the game's panel function.
1. In the GamePanel
class add a Timer
member variable called frameDraw. This is a new class that we haven't used yet. When importing the Timer
code, make sure you import the class that reads javax.swing.Timer
.
2. The timer is going to use the ActionListener
interface to make actions happen when it expires. Make your class implement the ActionListener
interface as shown below:
public class GamePanel extends JPanel implements ActionListener { ... }
Add the unimplemented method (Eclipse can help). You should now have an actionPerformed()
method in your class.
3. In the GamePanel
constructor, initialize its timer member variable. The constructor of the Timer class takes two parameters. The first parameter is an int for how fast your want the timer to repeat. This is in milliseconds so 1000 is equal to 1 second. We want the game to run at 60 frames per second. So the first parameter will be 1000 / 60. The second parameter takes a reference to an ActionListener object. Since we implemented it in the GamePanel
, we can put this for the second parameter. After you have initialized the variable, start the timer (see below).
frameDraw = new Timer(1000/60,this); frameDraw.start();
4. In the actionPerformed
method (which runs when the timer triggers it), add a series of if/else statements to check the currentState
variable. Call the appropriate update method inside the if/else block as shown.
if(currentState == MENU){ updateMenuState(); }else if(currentState == GAME){ updateGameState(); }else if(currentState == END){ updateEndState(); }
5. Add a line of code to the actionPerformed
method to print "action" to the console, followed by a call to the panel's repaint()
method to redraw the panel.
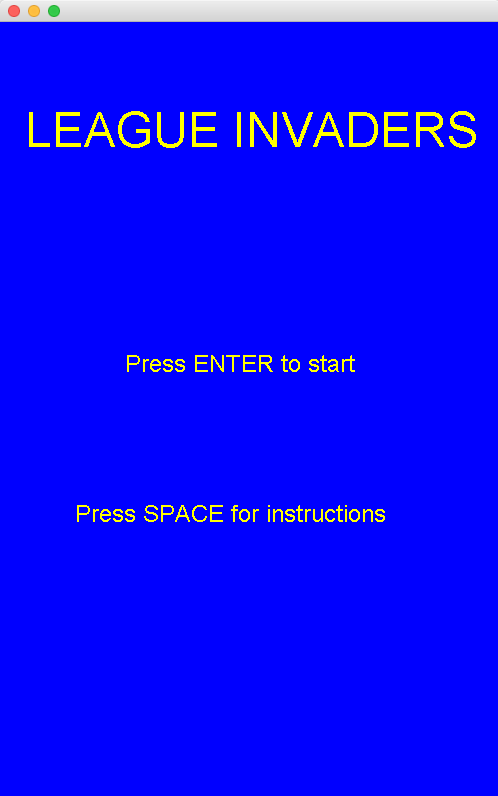
TESTING
6. Run the program. Is the menu frame displayed? Is "action" appearing repeatedly on the console? If not, get this part working before you move on to the next step.