Menu and End Game
drawing text
In order to draw text, we first have to make one or more Font objects. These will define the type, style, and size of the font used.
1. In the GamePanel
class, create a Font variable called titleFont
.
2. Create a constructor, and initialize the titleFont
variable as follows. The constructor of the Font class takes three parameters. The first is a String for the type of font you want to display. For now, set it to "Arial". The second parameter is for any stylization you would like to apply to the font. For now, set it to Font.PLAIN
. The third parameter is for the size of the font. Set it to 48 for now.
titleFont = new Font("Arial", Font.PLAIN, 48);
3. Go to the drawMenuState method and set the font for the graphics to use before drawing the menu text. Don't forget that you need to set the color of your text so that it will show up on your background color. E.g.
g.setFont(titleFont); g.setColor(Color.YELLOW); g.drawString("text", x, y);
4. Add text to the menu to match the League Invaders demo game. You may need to make more than one Font object to achieve this.
5. Add the text for the Game Over screen in the drawEndState method (you may need a different color). You will need to change this at the end of the game when you have implemented the code that keeps a score.
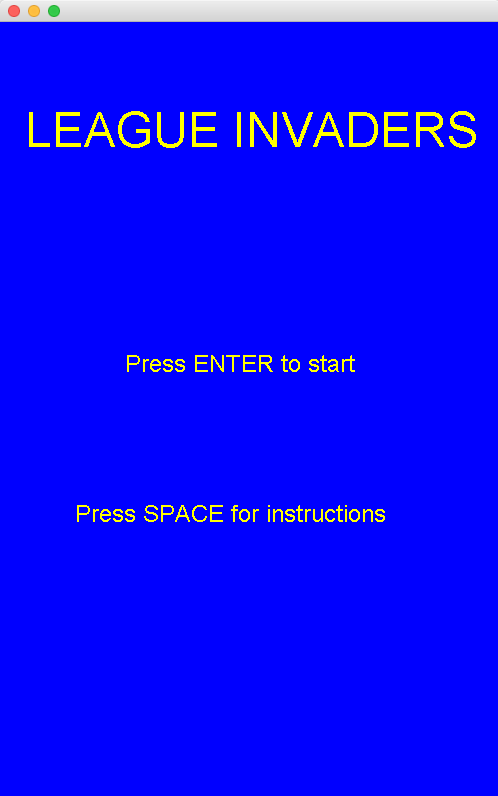
TESTING
6. Check the MENU window to make sure it has the correct appearance. We cannot check the END window until later.