League Invaders - Runner Class
1. Create a new class called LeagueInvaders. This will be the "runner" or "driver" class that controls the entire program using the other classes that you will create later.
2. Add a main method to this class. This will be the ONLY main method in the League Invaders program.
3. The game will be displayed in a JFrame. Create a member variable in your class that will hold the JFrame.
4. The size of the game window will remain constant and must be accessible to other objects in the game. Create two public static final int
variables for the WIDTH and the HEIGHT of the JFrame
. Note: By Java convention, these variable names are capitalized to indicate they will not change. Set the WIDTH to 500 and the HEIGHT to 800.
5. Create a constructor for the LeagueInvaders class that initializes the JFrame variable.
6. Create a void method called setup()
that will initialize the properties of the game window, including its size (set height and width using the variables created in step 4). This method also needs to make sure the JFrame shows up and also specifies:
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
7. Create a LeagueInvaders
object in your main method (i.e. call the constructor), and use this object to call the setup()
method.
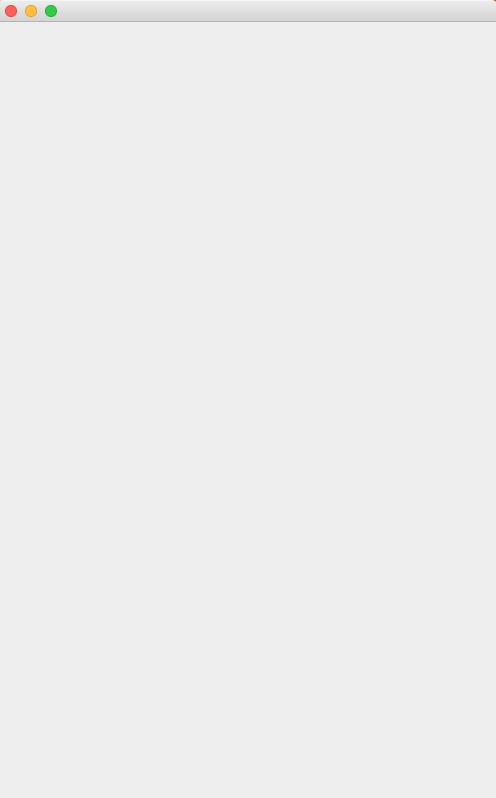
TESTING
8. Run the program. Is the game window displayed and is it the right size? If not, get this part working before you move on to the next step.