Rocket (draw)
All of the game characters share common characteristics. They all have to be drawn at a specific location (x,y) and have a specific size (width,height). The common characteristics will be stored in a "superclass" called GameObject.
GameObject
1. Create a new class called GameObject and add the following member variables to represent the information shared by all game characters:
int x; int y; int width; int height;
2. Make a constructor for this class that takes parameters to initialize all of the above member variables.
3. Add another int member variable called speed
and initialize it to 0 and a boolean member variable called isActive
and initialize it to true. These will be used later.
4. Make an empty update() method. Code will be added later, but you need to create the method now so other code will compile.
Rocketship
5. Create a Rocketship class that extends the GameObject class.
public class Rocketship extends GameObject { }
6. Add a constructor for this class that takes the same parameters as the inherited GameObject constructor (see step 2), and pass the parameters to the GameObject (superclass) constructor (see below). Note: Eclipse can generate this for you
super(x,y,width,height);
7. In the Rocketship
class, create a void draw()
method. The draw method takes a parameter that is a Graphics
object (g). For now we will draw a blue square to represent the rocketship by putting the following code in its draw()
method:
g.setColor(Color.BLUE); g.fillRect(x, y, width, height);
GamePanel
8. In the GamePanel
, create a new member variable to hold a Rocketship object that has x,y of 250,700 and width/height of 50,50.
9. In the drawGameState() method, add code to call the rocketship's draw method (pass it the Graphics object).
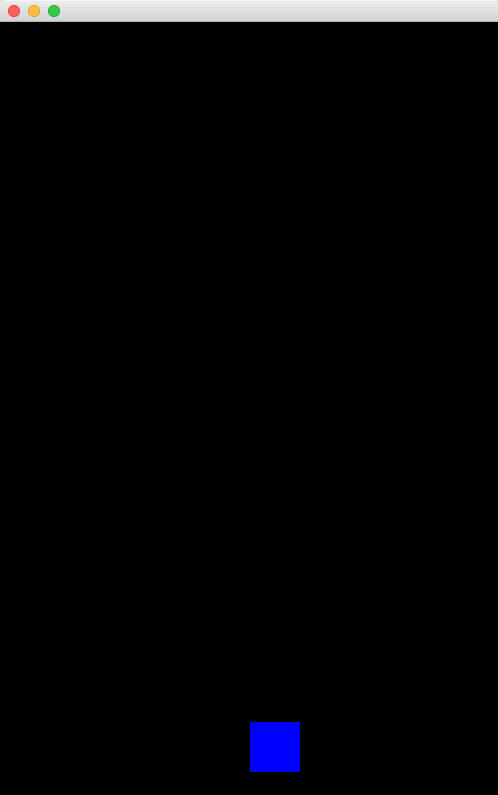
TESTING
9. Run the program. Press the Enter key to put the game into the GAME state (black background). Does the blue rocketship (square) appear at the bottom?