Model Management
Since the game characters share common properties, we can manage them via a single Object Manager. They will all have to be drawn, updated, and removed when they are no longer active.
ObjectManager
1. Create a new class called ObjectManager and add a rocket member variable
BUT DO NOT INITIALIZE IT
2. Make a constructor for this class that takes a Rocketship parameter and use it to initialize the member variable created above.
3. Add another member variable projectiles
that will hold an ArrayList of Projectile objects. Initialize this variable to an empty ArrayList. Add a void method addProjectile()
with a parameter that is a Projectile
object. This method needs to add the projectile to the ArrayList.
4. Add another member variable aliens
that will hold an ArrayList of Alien objects. Initialize this variable to an empty ArrayList. Add a member variable random
and initialize it to a new Random object. Also create an addAlien()
method that contains the code below.
aliens.add(new Alien(random.nextInt(LeagueInvaders.WIDTH),0,50,50));
5. Create a method called update()
. Iterate through all the aliens and call the update method on each alien. After updating, test the alien's y value. If it is outside the game window (use LeagueInvaders.HEIGHT), set the alien's isActive variable to false. Note: Iteration will require the use of a for loop of some kind! Next do a similar thing for all the projectiles.
6. Create a method called draw()
that has a Graphics parameter. Add code to call the rocket's draw method (pass it the graphics object). Then iterate through all the aliens and call the draw method on each. Now do the same thing for all the projectiles.
7. Create a method called purgeObjects()
. This method needs to iterate through all the aliens and projectiles and remove any that are marked as not active. Remember: You cannot use a for each loop when you are removing items from an ArrayList.
GamePanel
8. Inside the GamePanel
class, create and initialize a member varable for an ObjectManager
object. Use the panel's rocketship as the parameter for the ObjectManager constructor.
9. In the updateGameState
method, add a call to the objectManager's update method.
10. In the drawGameState
method, delete the call to the rocket's draw method and replace it with a call to the objectManager's draw method.
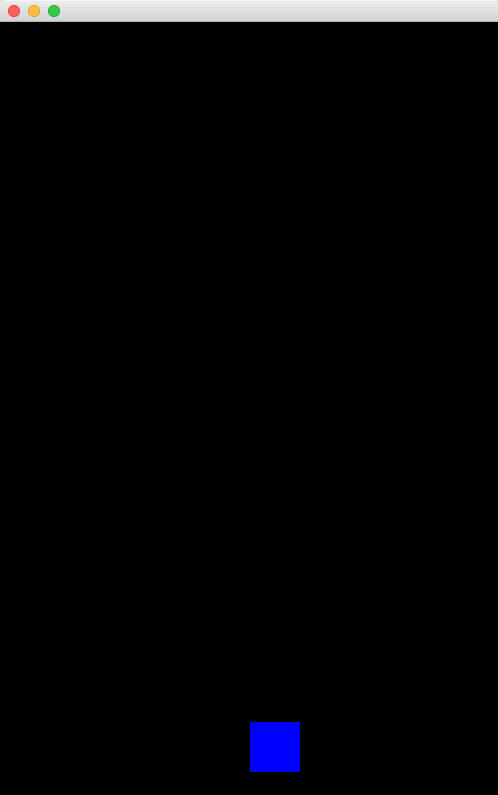
TESTING
11. Run the program. You should see no change from the previous test. That is, you should still see a blue rocket when in the GAME state, and your background color should still change when you press the Enter key.