Images
1. You can choose to use your own images for this game (they must be the correct size) or you can use the League images located in this folder:
- rocket.png
- alien.png
- bullet.png
- space.png
Place all the images you intend to use in the same package as the game source code.
Rocketship
2. Add these member variables:
public static BufferedImage image; public static boolean needImage = true; public static boolean gotImage = false;
3. Add this method that tries to load the image.
void loadImage(String imageFile) { if (needImage) { try { image = ImageIO.read(this.getClass().getResourceAsStream(imageFile)); gotImage = true; } catch (Exception e) { } needImage = false; } }
4. Call the loadImage method from the constructor:
if (needImage) { loadImage ("rocket.png"); }
5. In the draw method, draw the image if there is one, otherwise keep drawing the rectangle.
if (gotImage) { g.drawImage(image, x, y, width, height, null); } else { g.setColor(Color.BLUE); g.fillRect(x, y, width, height); }
TESTING
6. Run the program. Does the rocketship look better?
Alien and Projectile
7. Repeat steps 2 through 5 for the Alien and Projectile (bullet) images.
GamePanel
8. Draw the space image in place of the black rectangle for the background of the game.
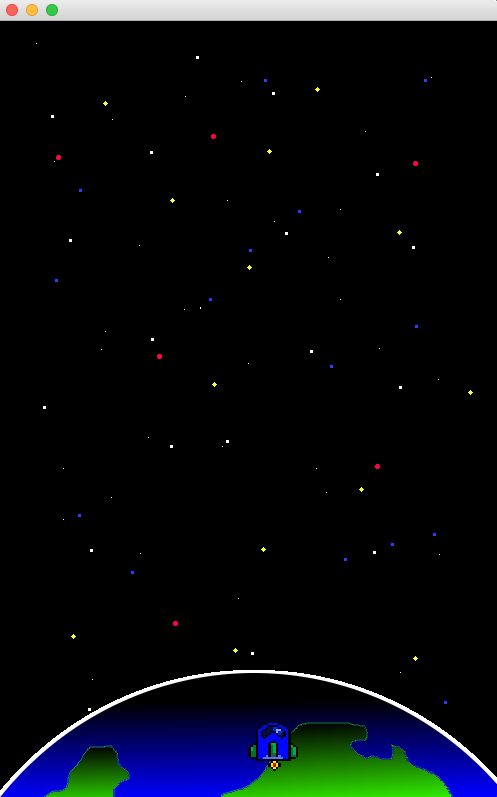
TESTING
9. Run the program. Does the background now look like space? Does the rocket image appear?