Alien Timer
A Timer will control the rate at which enemies will appear in the game. The following steps will create and start the timer as part of the GamePanel function, but the aliens will be added as part of the ObjetManager function.
ObjectManager
1. The ObjectManager
is going to use the ActionListener
interface to add an alien to the game each time it expires. Make the ObjectManager
class implement the ActionListener
interface as shown below:
public class ObjectManager implements ActionListener { ... }
Add the unimplemented method (Eclipse can help). You should now have an actionPerformed()
method in this class.
2. To make a new alien appear when the timer expires, call the addAlien() method:
addAlien();
Rocketship
3. Projectiles are launched from the rocket. Create the following method to make a projectile from the center of the rocket.
public Projectile getProjectile() { return new Projectile(x+width/2, y, 10, 10); }
GamePanel
4. In the GamePanel
class add another Timer
member variable called alienSpawn
. You should already have imported javax.swing.Timer
when you set up the main game timer. DO NOT INITIALIZE THIS VARIABLE YET.
5. We only want the aliens to be created while the game is running, so create a new method startGame()
. This method should initialize the alienSpawn
member variable and start this timer. The constructor of the Timer class takes two parameters. The first parameter is an int for how fast your want the timer to repeat in milliseconds. Let's start by having one alien spawn every second. So the first parameter will be 1000. The second parameter takes a reference to an ActionListener object. We are going to implement the alienSpawn code in the ObjectManager
, so we put objectManager as the second parameter (see below).
alienSpawn = new Timer(1000 , objectManager); alienSpawn.start();
6. Call the startGame() method when the game state changes from MENU to GAME (in the keyPressed() method). Also add code to stop this timer when the game state changes from GAME to END.
7. Add code to create new projectiles when the Space Bar is pressed in the GAME state. The ObjectManger has an addProjectile() method for this and the rocket will create the projectile in the correct location.
objectManager.addProjectile(rocket.getProjectile());
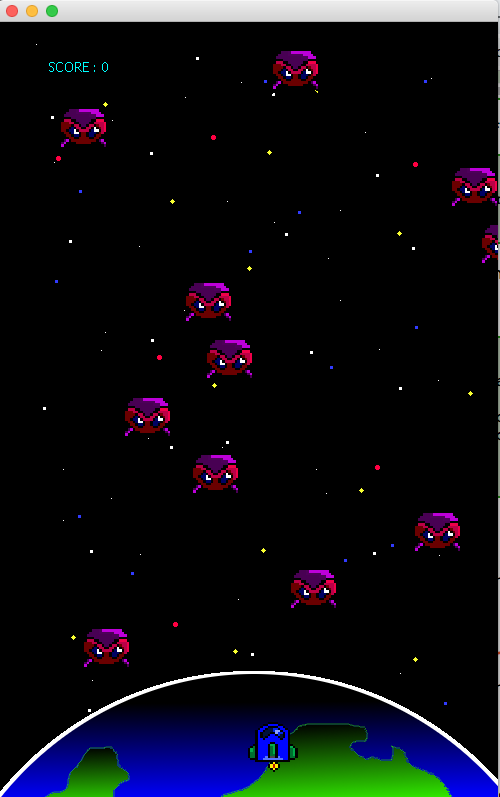
TESTING
8. Run the program. In the game state (space background) aliens should be falling from the top of the frame at a rate of about 1 per second. When you press the space bar, do projectiles go up from the rocket?