Alien
1. Create a Alien class that extends the GameObject class.
2. Add a constructor for this class that takes parameters to initialize all of the inherited GameObject member variables in the same way as you did for the Rocketship class. Set its speed variable to 1.
3. In the Alien
class, create 2 void methods, update()
and draw()
. In the draw method, draw a yellow rectangle using the following code:
g.setColor(Color.YELLOW); g.fillRect(x, y, width, height);
4. In the update()
method, add code to increment its y value by the value of speed (y+=speed
). This code will cause the Aliens to travel downwards as the game is played.
Projectile
5. Create a Projectile class that extends the GameObject class. Repeat steps 2 and 3 above, but in the constructor and set its speed variable to 10 and make the color of the rectangle RED in its draw method.
6. In the update()
method, add code to deccrement its y value by the value of speed (y-=speed
). This code will cause the Projectiles to travel upwards as the game is played.
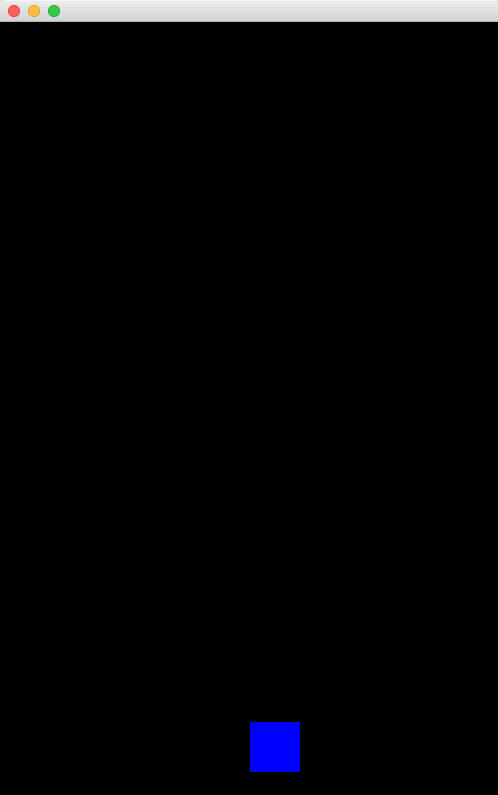
TESTING
7. Run the program. Press the Enter key to put the game into the GAME state (black background). Does the blue rocketship (square) still appear at the bottom? No other changes will appear yet as we have not yet added aliens or projectiles to the game panel.